I based a lot of it on Lee's bouncing points from earlier posts, but the mouse-proximity detection and ball-expanding is new. UPDATED with colour change built into the ball-expanding, and updated trails from ellipses to lines: they were a stream of trailing ellipses (size 1) but that meant the trails broke up at faster speeds, now replaced with lines.
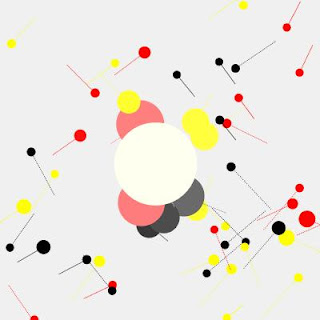
Ball[] myBall = new Ball[50];
void setup() {
size(400,400);
for (int i= 0; i < myBall.length; i++) {
int randCol = int(random(3));
myBall [i] = new Ball(randCol, 0, 0, random(0.5, 5), random(0.5, 5));
}
}
void draw() {
background(240);
for (int i= 0; i < myBall.length; i++) {
myBall[i].bounce();
myBall[i].display();
}
}
class Ball {
int c;
float xpos;
float ypos;
float xspeed;
float yspeed;
int i;
color col;
float [] holdX = new float[30];
float [] holdY = new float[30];
float big = 10;
Ball(int tempC, float tempXpos, float tempYpos, float tempXspeed, float tempYspeed) {
c = tempC;
xpos = tempXpos;
ypos = tempYpos;
xspeed = tempXspeed;
yspeed = tempYspeed;
}
void display() {
if (c == 0) {
col = color(1,1,1);
} else if (c == 1) {
col = color(255,0,0);
} else if (c == 2) {
col = color(255,255,0);
}
fill(col);
noStroke();
smooth();
int r = (col >> 16) & 0xFF; // Faster way of getting red(col)
int g = (col >> 8) & 0xFF; // Faster way of getting green(col)
int b = col & 0xFF; // Faster way of getting blue(col)
float rad = 100;
float kx = mouseX - xpos;
float ky = mouseY - ypos;
float mx = sqrt(pow(kx, 2) + pow(ky, 2));
if (mx <= rad) {
big = 10 + (100 - mx);
r = constrain(r + int(255 - 2.55*mx),0,255);
g = constrain(g + int(255 - 2.55*mx),0,255);
b = constrain(b + int(255 - 2.55*mx),0,255);
}
for (int i=0; i<29; i++) {
stroke(r,g,b);
line(holdX[i],holdY[i], holdX[i+1],holdY[i+1]);
}
fill(r,g,b);
ellipse(xpos,ypos, big, big);
for (int i=29; i>=1; i--) {
holdX[i] = holdX[i - 1];
holdY[i] = holdY[i - 1];
}
holdX[0] = xpos;
holdY[0] = ypos;
}
void bounce() {
xpos = xpos + xspeed;
if (xpos > width) {
xspeed = xspeed * -1;
}
if (xpos < 0) {
xspeed = xspeed * -1;
}
ypos = ypos - yspeed;
if (ypos > height) {
yspeed = yspeed * -1;
}
if (ypos < 0) {
yspeed = yspeed * -1;
}
}
}
void mousePressed() {
saveFrame("pic-####.jpg");
}